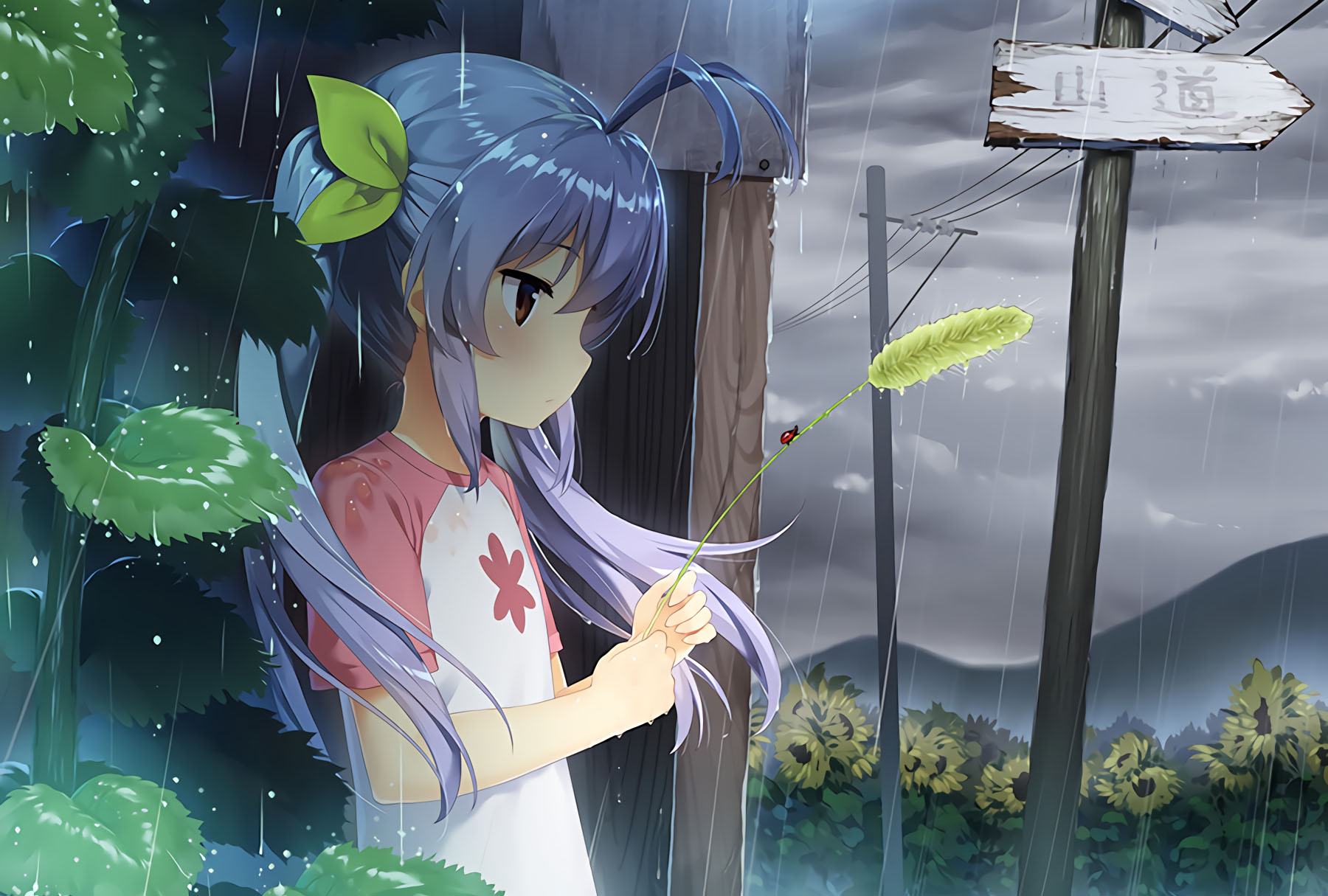
Vue3 vs Vue2
Vue3 vs Vue2
优势
组合式API:
- 更好的逻辑复用
- 更灵活的代码组织
- 更好的类型推导
- 更小的生产包体积
组合式API
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
| <script setup> import { ref, onMounted } from 'vue'
const count = ref(0)
function increment() { count.value++ }
onMounted(() => { console.log(`计数器初始值为 ${count.value}。`) }) </script>
<template> <button @click="increment">点击了:{{ count }} 次</button> </template>
|
全局API
1 2 3 4
| import { createApp } from 'vue'
const app = createApp({})
|
2.x 全局 API | 3.x 实例 API (app ) |
---|
Vue.config | app.config |
Vue.config.productionTip | 移除 (见下方) |
Vue.config.ignoredElements | app.config.compilerOptions.isCustomElement (见下方) |
Vue.component | app.component |
Vue.directive | app.directive |
Vue.mixin | app.mixin |
Vue.use | app.use (见下方) |
Vue.prototype | app.config.globalProperties (见下方) |
Vue.extend | 移除 (见下方) |
Treeshaking
1 2 3 4 5 6
| import { nextTick } from 'vue'
nextTick(() => { // 一些和 DOM 有关的东西 })
|
v-model 和 sync
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| // vue2 <ChildComponent v-model="pageTitle" />
<ChildComponent :value="pageTitle" @input="pageTitle = $event" />
<ChildComponent :title="pageTitle" @update:title="pageTitle = $event" /> <ChildComponent :title.sync="pageTitle" />
// vue3 <ChildComponent v-model="pageTitle" />
<ChildComponent :modelValue="pageTitle" @update:modelValue="pageTitle = $event" />
|
key
1 2 3 4 5 6 7 8 9 10 11
| <template v-for="item in list"> <div :key="'heading-' + item.id">...</div> <span :key="'content-' + item.id">...</span> </template>
<template v-for="item in list" :key="item.id"> <div>...</div> <span>...</span> </template>
|
v-if,v-for
2.x 版本中在一个元素上同时使用 v-if
和 v-for
时,v-for
会优先作用。
3.x 版本中 v-if
总是优先于 v-for
生效。
响应式原理
Vue 2 使用 getter / setters 完全是出于支持旧版本浏览器的限制。而在 Vue 3 中则使用了 Proxy 来创建响应式对象,仅将 getter / setter 用于 ref。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| function reactive(obj) { return new Proxy(obj, { get(target, key) { track(target, key) return target[key] }, set(target, key, value) { target[key] = value trigger(target, key) } }) }
function ref(value) { const refObject = { get value() { track(refObject, 'value') return value }, set value(newValue) { value = newValue trigger(refObject, 'value') } } return refObject }
|
track收集依赖,trigger触发更新